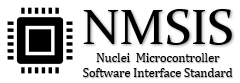 |
NMSIS-Core
Version 1.0.0-HummingBird
NMSIS-Core support for HummingBird RISC-V processor-based devices
|
28 #include "hbird_sdk_hal.h"
36 #define SYSTEM_CLOCK (80000000UL)
139 #define MAX_SYSTEM_EXCEPTION_NUM 11
156 typedef void (*
EXC_HANDLER) (
unsigned long mcause,
unsigned long sp);
157 typedef void (*
INT_HANDLER) (
unsigned long mcause,
unsigned long sp);
170 printf(
"Trap in Exception\r\n");
171 printf(
"MCAUSE: 0x%lx\r\n", mcause);
185 printf(
"Trap in Interrupt\r\n");
186 printf(
"MCAUSE: 0x%lx\r\n", mcause);
215 for (i = 0; i < __PLIC_INTNUM; i++) {
218 for (i = 0; i < 10; i++) {
246 if ((irqn <= 10) && (irqn >= 0)) {
260 if ((irqn <= __PLIC_INTNUM) && (irqn >= 0)) {
273 if ((irqn <= 10) && (irqn >= 0)) {
287 if ((irqn <= __PLIC_INTNUM) && (irqn >= 0)) {
325 uint32_t irqn = (uint32_t)(mcause & 0X00000fff);
327 #if defined(__PLIC_PRESENT) && __PLIC_PRESENT == 1
329 if (irqn < __PLIC_INTNUM) {
331 if (int_handler != NULL) {
332 int_handler(mcause, sp);
339 if (int_handler != NULL) {
340 int_handler(mcause, sp);
362 uint32_t EXCn = (uint32_t)(mcause & 0X00000fff);
370 if (exc_handler != NULL) {
371 exc_handler(mcause, sp);
380 #if defined(HBIRD_BANNER) && (HBIRD_BANNER == 1)
381 printf(
"HummingBird SDK Build Time: %s, %s\r\n", __DATE__, __TIME__);
382 #ifdef DOWNLOAD_MODE_STRING
383 printf(
"Download Mode: %s\r\n", DOWNLOAD_MODE_STRING);
405 if (handler != NULL) {
423 #if defined(__PLIC_PRESENT) && __PLIC_PRESENT == 1
437 if ((source >= __PLIC_INTNUM)) {
443 if (handler != NULL) {
463 void _premain_init(
void)
467 #if ! defined(SIMULATION_SPIKE) && ! defined(SIMULATION_XLSPIKE)
468 gpio_iof_config(GPIO, IOF0_UART0_MASK, IOF_SEL_0);
469 uart_init(SOC_DEBUG_UART, 115200);
477 #if defined(__PLIC_PRESENT) && __PLIC_PRESENT == 1
491 void _postmain_fini(
int status)
494 #ifdef SIMULATION_XLSPIKE
495 extern void xlspike_exit(
int status);
496 xlspike_exit(status);
498 #ifdef SIMULATION_SPIKE
499 extern void spike_exit(
int status);
@ SysTimer_IRQn
System Timer Interrupt.
void SystemBannerPrint(void)
Banner Print for HummingBird SDK.
__STATIC_FORCEINLINE void PLIC_Init(uint32_t num_sources)
Perform init for plic of current hart.
__STATIC_FORCEINLINE void __enable_timer_irq(void)
Enable Timer IRQ Interrupts.
unsigned long Interrupt_Get_ExtIRQ(uint32_t irqn)
Get an external interrupt handler for external interrupt number.
__STATIC_FORCEINLINE void __enable_sw_irq(void)
Enable software IRQ Interrupts.
static void system_default_exception_handler(unsigned long mcause, unsigned long sp)
System Default Exception Handler.
static unsigned long SystemCoreInterruptHandlers[10]
void SystemCoreClockUpdate(void)
Function to update the variable SystemCoreClock.
void(* INT_HANDLER)(unsigned long mcause, unsigned long sp)
__STATIC_FORCEINLINE void PLIC_EnableInterrupt(uint32_t source)
Enable interrupt for selected source plic.
static unsigned long SystemExceptionHandlers[MAX_SYSTEM_EXCEPTION_NUM]
Store the exception handlers for each exception ID.
#define MAX_SYSTEM_EXCEPTION_NUM
Max exception handler number.
__STATIC_FORCEINLINE void __enable_ext_irq(void)
Enable External IRQ Interrupts.
uint32_t SystemCoreClock
Variable to hold the system core clock value.
void Exception_Register_EXC(uint32_t EXCn, unsigned long exc_handler)
Register an exception handler for exception code EXCn.
void Interrupt_Register_ExtIRQ(uint32_t irqn, unsigned long int_handler)
Register an external interrupt handler for plic external interrupt number.
static void Exception_Init(void)
Initialize all the default core exception handlers.
void(* EXC_HANDLER)(unsigned long mcause, unsigned long sp)
Exception Handler Function Typedef.
int32_t PLIC_Register_IRQ(uint32_t source, uint8_t priority, void *handler)
Register a specific plic interrupt and register the handler.
unsigned long Interrupt_Get_CoreIRQ(uint32_t irqn)
Get an core interrupt handler for core interrupt number.
static void Interrupt_Init(void)
Initialize all the default interrupt handlers.
#define __RV_CSR_READ(csr)
CSR operation Macro for csrr instruction.
static unsigned long SystemExtInterruptHandlers[__PLIC_INTNUM]
void SystemInit(void)
Function to Initialize the system.
int32_t Core_Register_IRQ(uint32_t irqn, void *handler)
Register a riscv core interrupt and register the handler.
uint32_t core_trap_handler(unsigned long mcause, unsigned long sp)
Common trap entry.
__STATIC_FORCEINLINE void PLIC_CompleteInterrupt(uint32_t source)
Complete interrupt for plic of current hart.
unsigned long Exception_Get_EXC(uint32_t EXCn)
Get current exception handler for exception code EXCn.
static void system_default_interrupt_handler(unsigned long mcause, unsigned long sp)
System Default Interrupt Handler.
__STATIC_FORCEINLINE uint32_t PLIC_ClaimInterrupt(void)
Claim interrupt for plic of current hart.
@ SysTimerSW_IRQn
System Timer SW interrupt.
__STATIC_FORCEINLINE void PLIC_SetPriority(uint32_t source, uint32_t priority)
Set interrupt priority for selected source plic.
void Interrupt_Register_CoreIRQ(uint32_t irqn, unsigned long int_handler)
Register an core interrupt handler for core interrupt number.
static uint32_t core_exception_handler(unsigned long mcause, unsigned long sp)
Common Exception handler entry.