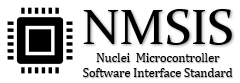 |
NMSIS-Core
Version 1.0.0-HummingBird
NMSIS-Core support for HummingBird RISC-V processor-based devices
|
19 #ifndef __NMSIS_GCC_H__
20 #define __NMSIS_GCC_H__
26 #include "riscv_encoding.h"
46 #pragma GCC diagnostic push
47 #pragma GCC diagnostic ignored "-Wsign-conversion"
48 #pragma GCC diagnostic ignored "-Wconversion"
49 #pragma GCC diagnostic ignored "-Wunused-parameter"
53 #define __has_builtin(x) (0)
64 #define __INLINE inline
68 #ifndef __STATIC_INLINE
69 #define __STATIC_INLINE static inline
73 #ifndef __STATIC_FORCEINLINE
74 #define __STATIC_FORCEINLINE __attribute__((always_inline)) static inline
79 #define __NO_RETURN __attribute__((__noreturn__))
84 #define __USED __attribute__((used))
89 #define __WEAK __attribute__((weak))
94 #define __VECTOR_SIZE(x) __attribute__((vector_size(x)))
99 #define __PACKED __attribute__((packed, aligned(1)))
103 #ifndef __PACKED_STRUCT
104 #define __PACKED_STRUCT struct __attribute__((packed, aligned(1)))
108 #ifndef __PACKED_UNION
109 #define __PACKED_UNION union __attribute__((packed, aligned(1)))
112 #ifndef __UNALIGNED_UINT16_WRITE
113 #pragma GCC diagnostic push
114 #pragma GCC diagnostic ignored "-Wpacked"
115 #pragma GCC diagnostic ignored "-Wattributes"
120 #pragma GCC diagnostic pop
122 #define __UNALIGNED_UINT16_WRITE(addr, val) (void)((((struct T_UINT16_WRITE *)(void *)(addr))->v) = (val))
125 #ifndef __UNALIGNED_UINT16_READ
126 #pragma GCC diagnostic push
127 #pragma GCC diagnostic ignored "-Wpacked"
128 #pragma GCC diagnostic ignored "-Wattributes"
133 #pragma GCC diagnostic pop
135 #define __UNALIGNED_UINT16_READ(addr) (((const struct T_UINT16_READ *)(const void *)(addr))->v)
138 #ifndef __UNALIGNED_UINT32_WRITE
139 #pragma GCC diagnostic push
140 #pragma GCC diagnostic ignored "-Wpacked"
141 #pragma GCC diagnostic ignored "-Wattributes"
146 #pragma GCC diagnostic pop
148 #define __UNALIGNED_UINT32_WRITE(addr, val) (void)((((struct T_UINT32_WRITE *)(void *)(addr))->v) = (val))
151 #ifndef __UNALIGNED_UINT32_READ
152 #pragma GCC diagnostic push
153 #pragma GCC diagnostic ignored "-Wpacked"
154 #pragma GCC diagnostic ignored "-Wattributes"
159 #pragma GCC diagnostic pop
161 #define __UNALIGNED_UINT32_READ(addr) (((const struct T_UINT32_READ *)(const void *)(addr))->v)
166 #define __ALIGNED(x) __attribute__((aligned(x)))
171 #define __RESTRICT __restrict
175 #ifndef __COMPILER_BARRIER
176 #define __COMPILER_BARRIER() __ASM volatile("":::"memory")
181 #define __USUALLY(exp) __builtin_expect((exp), 1)
186 #define __RARELY(exp) __builtin_expect((exp), 0)
214 #define __I volatile const
219 #define __IO volatile
223 #define __IM volatile const
225 #define __OM volatile
227 #define __IOM volatile
242 #define _VAL2FLD(field, value) (((uint32_t)(value) << field ## _Pos) & field ## _Msk)
257 #define _FLD2VAL(field, value) (((uint32_t)(value) & field ## _Msk) >> field ## _Pos)
#define __PACKED_STRUCT
Request smallest possible alignment for a structure.
__PACKED_STRUCT T_UINT32_WRITE
Packed struct for unaligned uint32_t write access.
__PACKED_STRUCT T_UINT32_READ
Packed struct for unaligned uint32_t read access.
__PACKED_STRUCT T_UINT16_WRITE
Packed struct for unaligned uint16_t write access.
__PACKED_STRUCT T_UINT16_READ
Packed struct for unaligned uint16_t read access.